Python Basic - 1
Everything in Python is an Object
How to Format Floats Within F-Strings in Python
Arithmetic, Boolean and Comparison Operators
Cheat Sheets (Matplotlib and others)
Procedural Programming Style versus Object-Oriented
​
How to Define a Function in Python
Methods
Create Python Modules (aka Packages or Libraries)
​
More information about "arrays"
Numpy dtype objects
Array Broadcasting in Numpy
Vectorized Code
3D Graph in Python
​
pandas (https://pandas.pydata.org/)
pandas - retrieving information
pandas - dataframe.dropna(...)
pandas - dataframe.drop(...)
pandas - dataframe.rank(...)
How to Create Pivot Tables with pandas – Real Python - may 2024
Pandas Cheat Sheet for Data Science in Python – DataCamp - july 2024
Everything in Python is a Object

Michael T. Goodrich , Roberto Tamassia and Michael H. Goldwasser,
“Data Structures and Algorithms in Python”, 1st Edition , Wiley, March 18, 2013.
How to Format Floats Within F-Strings in Python - Real Python - apr 2024
# prompt: please generate a python code with comma print 1486982323.6787
number = 1486982323.6787
print(f"number here = {number:,.2f}")
# prompt: please generate a python code with comma print
for i in range(project_lifetime):
print(f'Ano {i + 1}: Receita = {revenues[i]:,.2f}, Custo = {costs[i]:,.2f}, Fluxo de Caixa = {cash_flows[i]:,.2f}')
#end for
Arithmetic, Boolean and Comparison Operators

Cheat Sheets (Matplotlib and others)
Matplotlib for Beginners
Matplotlib for Intermediate
Procedural Programming Style versus Object-Oriented
Introductory courses and books typically teach software development using the procedural programming style, which involves splitting a program into a number of functions (also known as procedures or subroutines). You pass data into functions, each of which performs one or more computations and, typically, passes back results.
This book is about a different paradigm of programming known as object-oriented programming (OOP) that allows programmers to think differently about how to build software. Object-oriented programming gives programmers a way to combine code and data together into cohesive units, thereby avoiding some complications inherent in procedural programming.
Irv Kalb , “Object-Oriented Python: Master OOP by Building Games and GUIs”,
No Starch Press, January 25, 2022.
How to Define a Function in Python
When you write code that calls function len(), you don’t care how len() does what it does. You don’t stop to think about whether the code of the function contains two lines or two thousand lines, whether it uses one local variable or a hundred. You just need to know what argument to pass in and how to use the result that’s returned.
The same is true of functions that you write, like this function that calculates and returns the average of a list of numbers:
_____________________________________________________________
def calculateAverage(numbersList):
total = 0.0
for number in numbersList:
total = total + number
nElements = len(numbersList)
average = total / nElements
return average
___________________________________________________________
Once you’ve tested a function like this and found that it works, you no longer have to worry about the details of the implementation. You only need to know what argument(s) to send into the function and what it returns.
However, if one day you find that there is a much simpler or faster algorithm to calculate an average, you could rewrite the function in a new way. As long as the interface (the inputs and outputs) does not change, there is no need to change any calls to the function. This type of modularization makes the code more maintainable.
Irv Kalb , “Object-Oriented Python: Master OOP by Building Games and GUIs”,
No Starch Press, January 25, 2022.
Methods
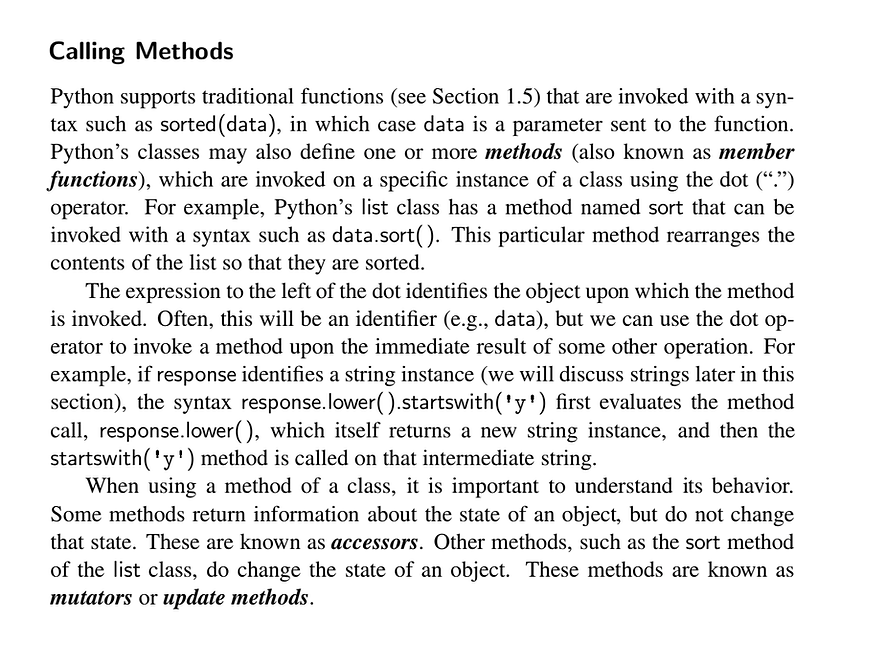
Michael T. Goodrich , Roberto Tamassia and Michael H. Goldwasser,
“Data Structures and Algorithms in Python”, 1st Edition , Wiley, March 18, 2013.
Create Python Modules (aka Packages or Libraries)

More information about "arrays"
An array is usually a fixed-size container of items of the same type and size. The number of dimensions and items in an array is defined by its shape. The shape of an array is a tuple of non-negative integers that specify the sizes of each dimension.
In NumPy, dimensions are called axes. This means that if you have a 2D array that looks like this:
array1 = [[0., 0., 0.],
[1., 1., 1.]]
Your array has 2 axes. The first axis has a length of 2 and the second axis has a length of 3.
A primeira dimensão é a linha e a segunda dimensão é a coluna!!!
You might occasionally hear an array referred to as a “ndarray,” which is shorthand for “N-dimensional array.” An N-dimensional array is simply an array with any number of dimensions. You might also hear 1-D, or one-dimensional array, 2-D, or two-dimensional array, and so on. The NumPy ndarray class is used to represent both matrices and vectors. A vector is an array with a single dimension (there’s no difference between row and column vectors), while a matrix refers to an array with two dimensions. For 3-D or higher dimensional arrays, the term tensor is also commonly used.
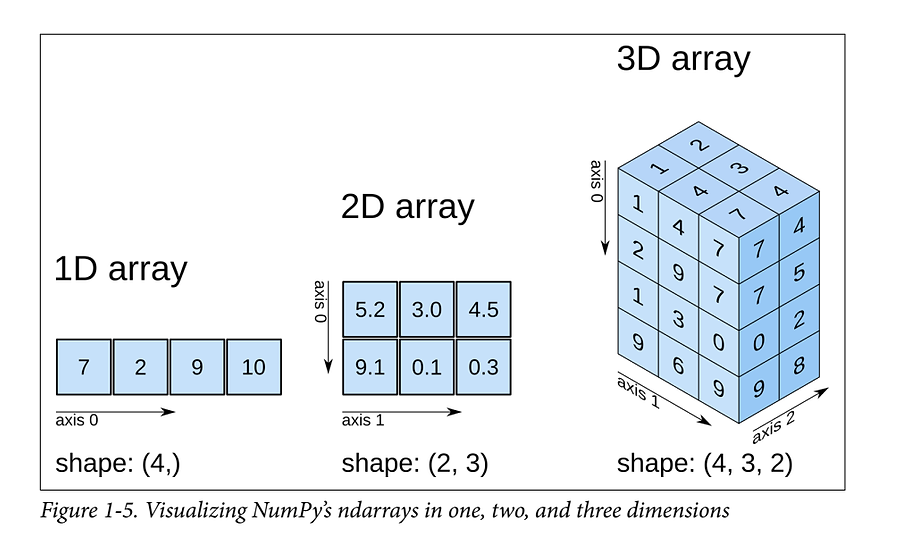
Juan Nunez-Iglesias, Stéfan van der Walt and Harriet Dashnow,
“Elegant SciPy: The Art of Scientific Python”,
O'Reilly Media, 1st Edition, September 26, 2017.
Numpy dtype objects
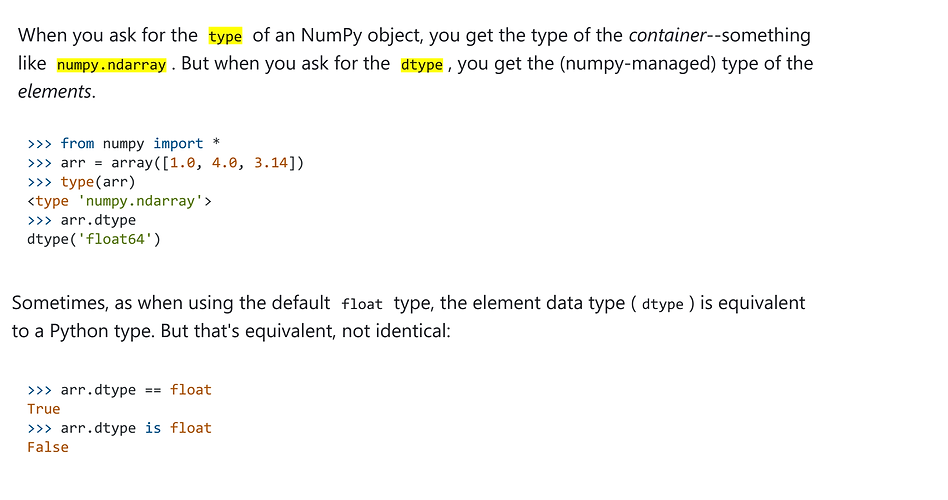

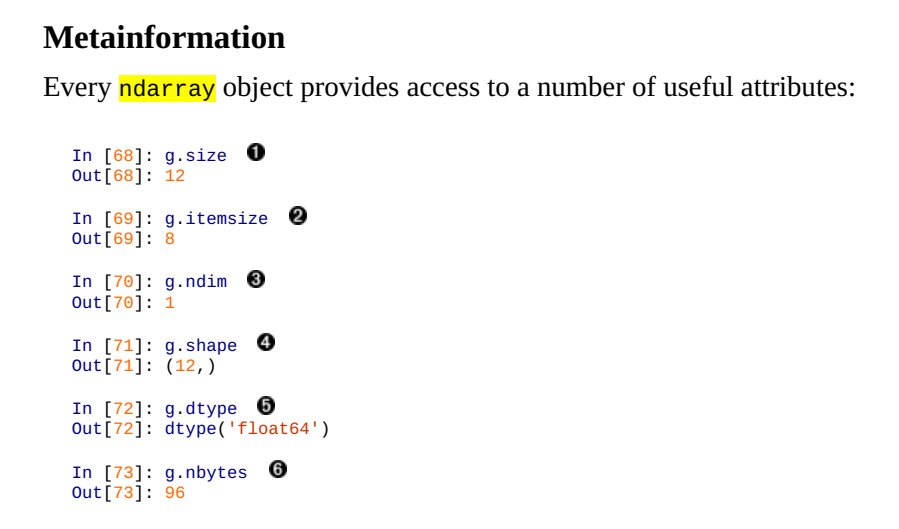
Array Broadcasting in Numpy
Let’s explore a more advanced concept in numpy called broadcasting. The term broadcasting describes how numpy treats arrays with different shapes during arithmetic operations. Subject to certain constraints, the smaller array is “broadcast” across the larger array so that they have compatible shapes. Broadcasting provides a means of vectorizing array operations so that looping occurs in C instead of Python. It does this without making needless copies of data and usually leads to efficient algorithm implementations.
There are also cases where broadcasting is a bad idea because it leads to inefficient use of memory that slows computation. This article provides a gentle introduction to broadcasting with numerous examples ranging from simple to involved. It also provides hints on when and when not to use broadcasting.
numpy operations are usually done element-by-element which requires two arrays to have exactly the same shape.
Vectorized Code
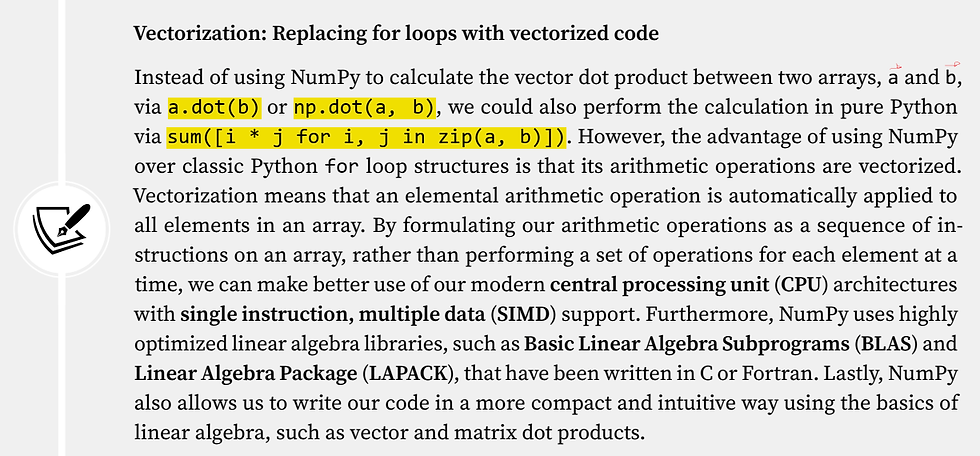
Sebastian Raschka, Yuxi (Hayden) Liu, Vahid Mirjalili, “Machine Learning with PyTorch and Scikit-Learn: Develop machine learning and deep learning models with Python”, Packt Publishing, February 25, 2022.
3D Graph in Python
# importing libraries
import numpy as np
import matplotlib.pyplot as plt
# defining surface and axes
number_of_points = 40
x = np.outer(np.linspace(-5, 5, number_of_points), np.ones(number_of_points))
y = x.copy().T
var1 = (x**2) + (y**2)
var2 = np.sin(np.sqrt(var1))
var3 = var2**2
var4 = (var3 - 0.5) / (1.0 + (0.1*var1))
z = 0.5 + var4
fig = plt.figure()
# syntax for 3-D plotting
ax = plt.axes(projection='3d')
# syntax for plotting
ax.plot_surface(x, y, z, cmap='viridis',\
edgecolor='green')
ax.set_title('Surface plot geeks for geeks')
plt.show()
pandas (https://pandas.pydata.org/)
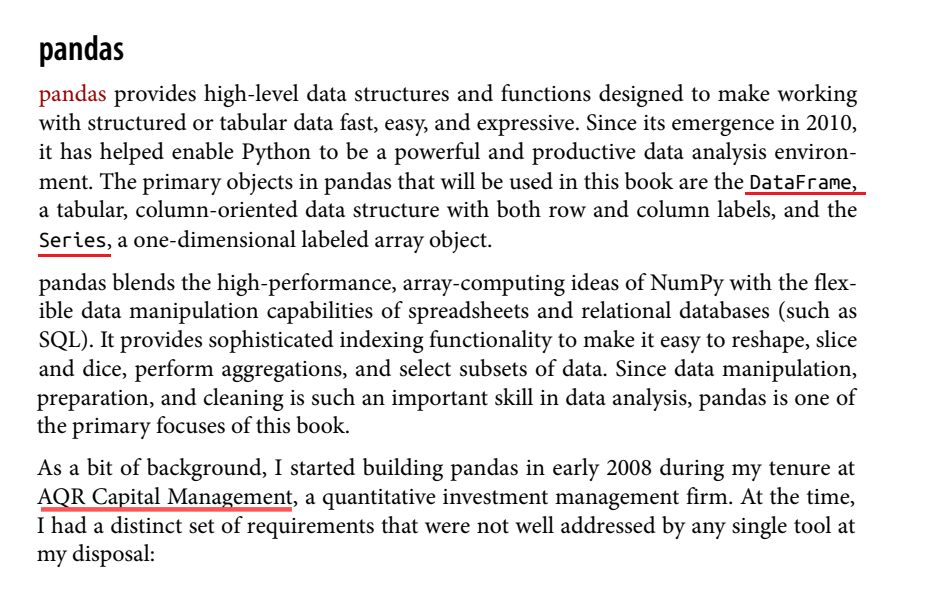
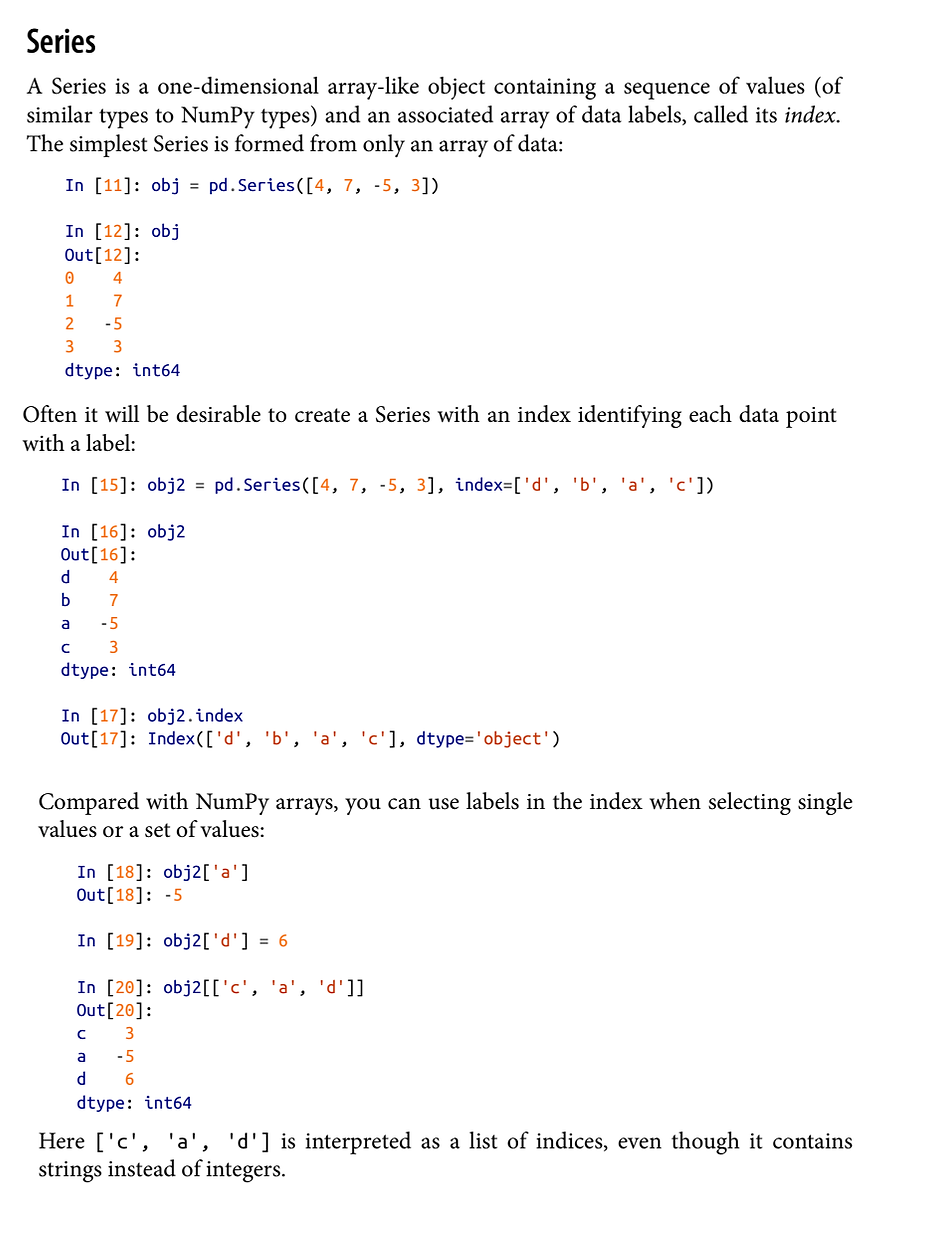
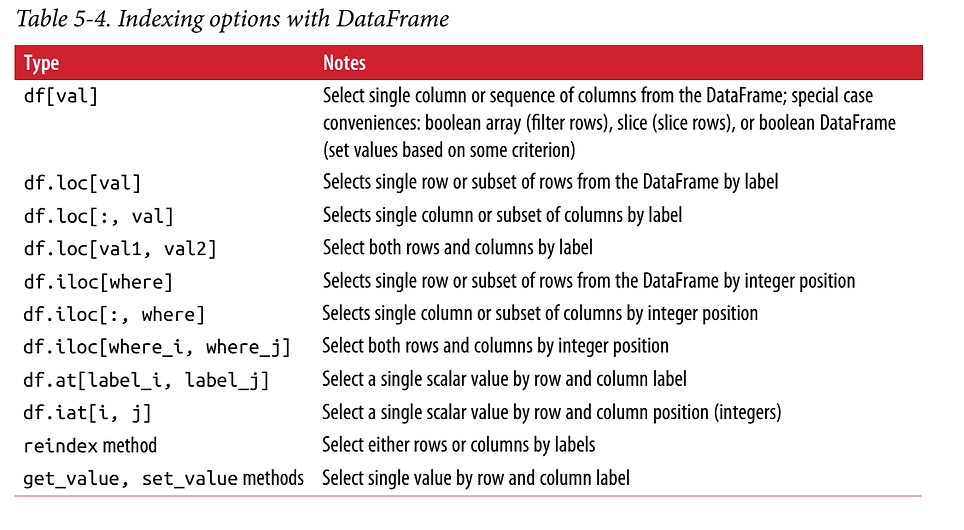
Wes McKinney, "Python for Data Analysis: Data Wrangling with Pandas, NumPy, and IPython", 2nd Edition, Publisher: O'Reilly Media, October 31, 2017.
pandas - retrieving information
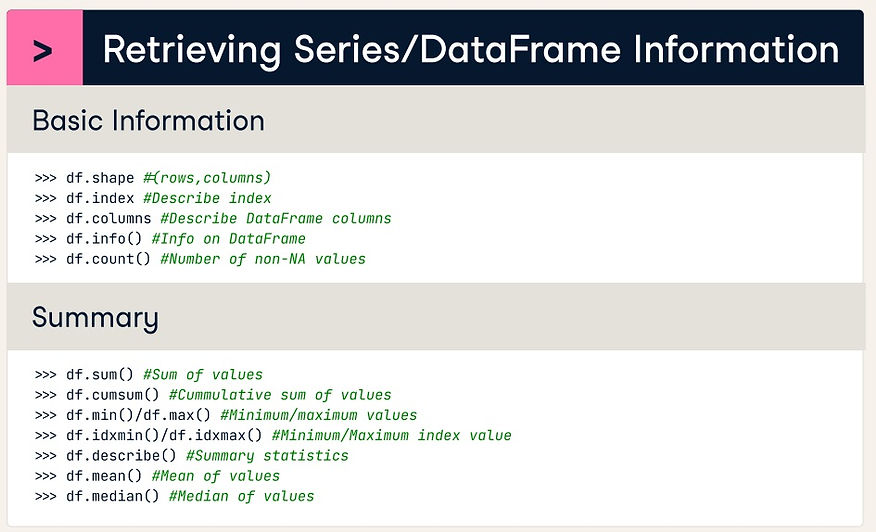
pandas - dataframe.dropna(...)
Remove all rows wit NULL values from the DataFrame.
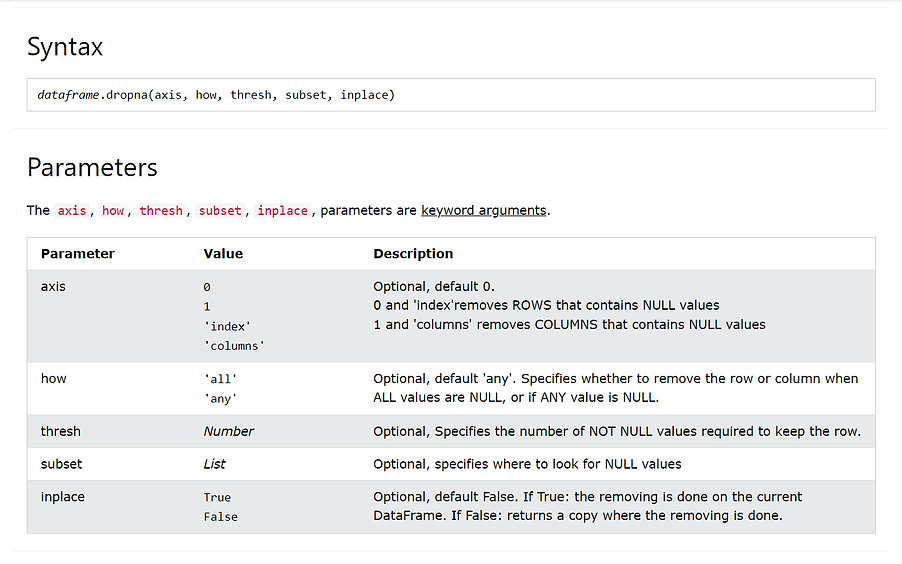
pandas - dataframe.drop(...)
Remove rows or columns by specifying label names and corresponding axis, or by directly specifying index or column names.

pandas - dataframe.rank(...)
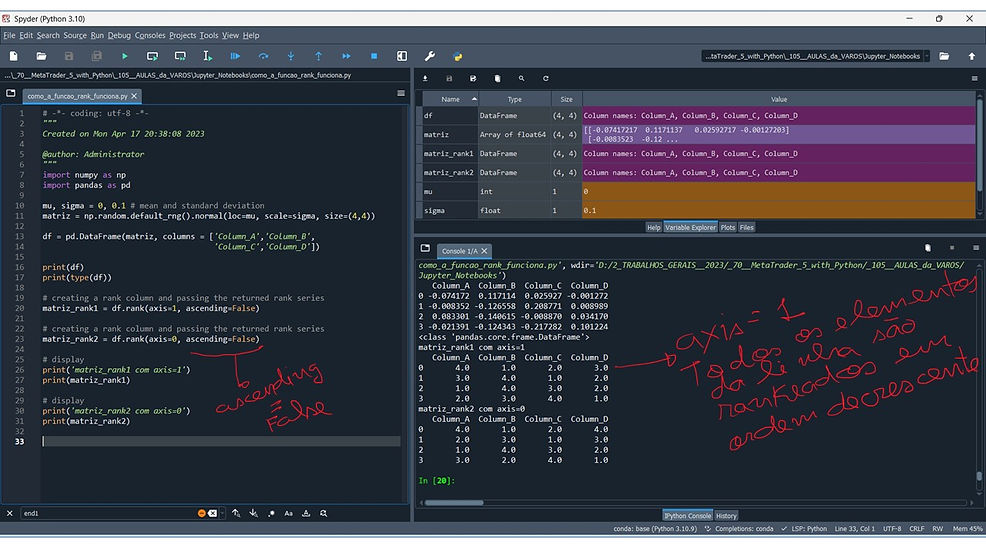